Uncover Hidden Treasures in Deeply Nested Objects with JavaScript
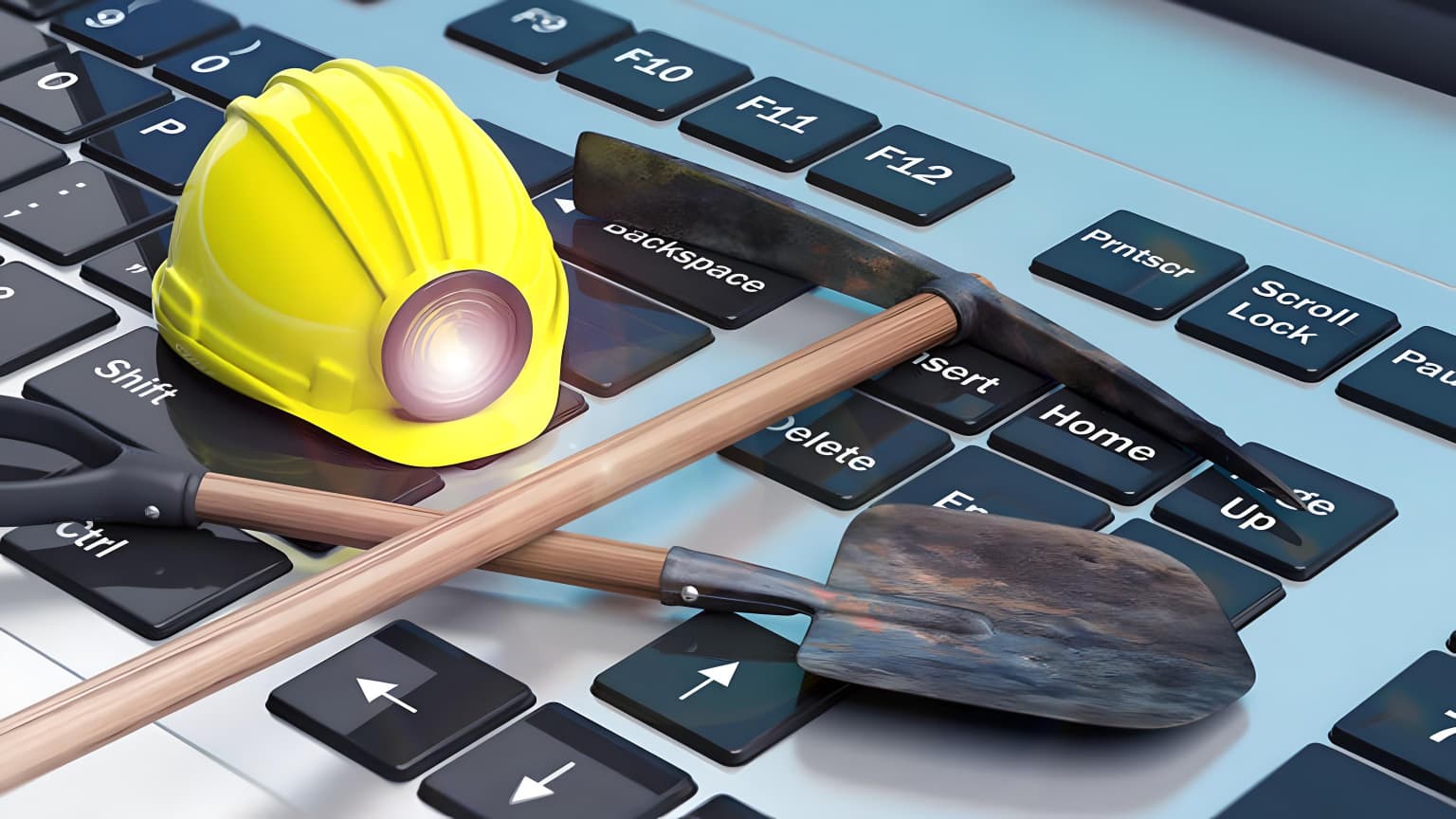
Uncover Hidden Treasures in Deeply Nested Objects with JavaScript
Do you find yourself lost in the labyrinth of nested JavaScript objects, desperately seeking that one elusive key? You're not alone! Nested objects can be tricky to navigate, but fear not; we've got a solution for you. In this article, we'll dive into the world of deep nested objects and explore two handy methods: find
and findAll
, which can help you unearth the hidden gems within.
The Deep Nesting Conundrum
Imagine you have a complex JavaScript object like this:
...
const deepNestedObject = {
level1: {
stringKey: "Hello, World!",
numberKey: 42,
booleanKey: true,
nullKey: null,
undefinedKey: undefined,
objectKey: {
level2: {
arrayKey: [1, 2, 3],
dateKey: new Date(),
functionKey: function () {
return `I am a function!`;
},
arrowFunctionKey: () => "I am a function!",
finalKey: "Found 2 level deep!",
regexKey: /pattern/,
symbolKey: Symbol("symbol"),
mapKey: new Map([
["mapKey1", "mapValue1"],
["mapKey2", "mapValue2"],
]),
level3: {
nestedObject: {
level4: {
deeplyNested: {
level5: {
level6: {
level7: {
finalKey: "This is the deepest level!",
},
},
},
},
},
},
},
},
},
},
};
...
You know there's valuable data hidden deep inside, but how do you access it without getting lost in the layers of nesting? This is where the find
and findAll
methods come to the rescue.
The find Method
The find
method is like a treasure map for your nested objects. It helps you find the first occurrence of a specified key, no matter how deeply it's buried. Let's take a closer look at how it works:
Object.prototype.find = function (key) {
if (typeof key !== "string" || key === "") return null;
const stack = [this];
while (stack.length > 0) {
const obj = stack.pop();
if (obj === null || typeof obj !== "object") continue;
const keys = Object.keys(obj);
for (const k of keys) {
if (k === key) {
return obj[k];
} else if (typeof obj[k] === "object") {
stack.push(obj[k]);
}
}
}
return null;
};
...
...
{
// find-Result, For Existing Key
const result = deepNestedObject.find("finalKey");
console.log(result); // Found 2 level deep!
}
{
// find-Result, For Non Existing Key
const findResultForNonExistingKey = deepNestedObject.find("nonExistentKey");
console.log(findResultForNonExistingKey); // null
}
...
The findAll Method
But what if there are multiple occurrences of the same key? The findAll method comes to your rescue! It's like a metal detector that uncovers all instances of the specified key within the nested object:
...
Object.prototype.findAll = function (key, defaultValue = []) {
if (typeof key !== "string" || key === "") return null;
const stack = [this];
const foundValues = [];
while (stack.length > 0) {
const obj = stack.pop();
if (obj === null || typeof obj !== "object") continue;
const keys = Object.keys(obj);
for (const k of keys) {
if (k === key) {
foundValues.push(obj[k]);
} else if (typeof obj[k] === "object") {
stack.push(obj[k]);
}
}
}
return foundValues.length > 0 ? foundValues : defaultValue;
};
...
...
{
// findAll-Result, For Existing Key
const result = deepNestedObject.findAll("finalKey");
console.log(result); // [ 'Found 2 level deep!', 'This is the deepest level!' ]
}
{
// findAll-Result, For Non Existing Key
const result = deepNestedObject.findAll("nonExistentKey");
console.log(result); // []
}
{
// findAll-Result, For Non Existing Key And Passing Second Parameter As Default Value
const result = deepNestedObject.findAll("nonExistentKey", null);
console.log(result); // null
}
The findAll method tirelessly searches every nook and cranny of your nested object, ensuring you don't miss a single treasure trove of data.
Conclusion
Navigating deeply nested JavaScript objects no longer has to be a daunting task. With the find and findAll methods at your disposal, you can confidently explore and extract valuable information from even the most intricate data structures. So go ahead, dive into those nested objects and uncover the hidden treasures that await you! Happy coding!
Check out the complete code here in the GitHub repository!